This is part 2 of building a RESTful API with Node.js, Express and ECMAScript 6 using a module approach. Be sure to read part 1 first.
Go to https://github.com/LuukMoret/blog-node-api-express-es6-part2 to see the finished code.
Now that the project setup is done we can start writing our own modules.
Create dummies module
Create dummies folder inside the app folder
mkdir app/dummies
Create controller file dummies.controller.js
inside app/dummies
, this will handle the routing of the module. Add the following content:
|
Update middleware/routes.js
file by adding the newly created route. Replace the file with the following content:
|
Create service file dummies.service.js
inside app/dummies
, this will contain the (business) logic of the module. Add the following content:
|
Create model file dummies.model.js
inside app/dummies
, this will handle data transformation. Add the following content:
|
Create repository file dummies.repository.js
inside app/dummies
, this will handle the repository calls (in this case git api call for demo purposes). Add the following content:
|
We can test the application now. Run npm start
and navigate with a browser to http://localhost:3100/dummies and you should see a json response from the node application.
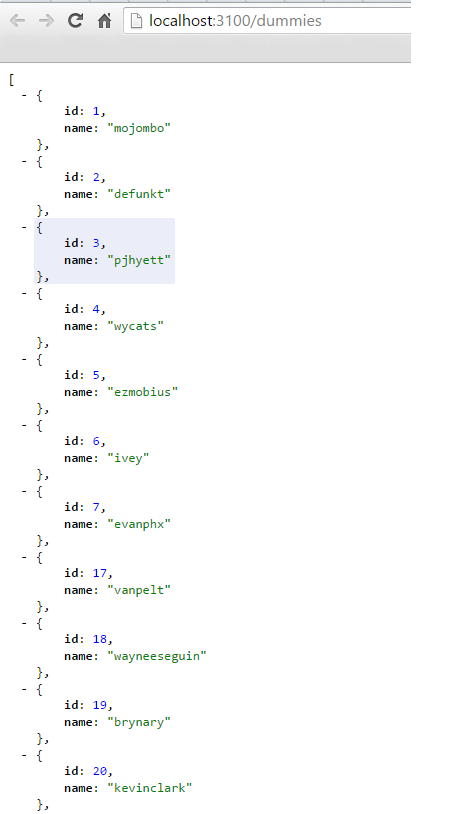
Now that we have written our dummies module it is time to write some unit and integration tests.
Create controller spec file dummies.controller.spec.js
inside app/dummies
with the following content:
|
Create service spec file dummies.service.spec.js
inside app/dummies
with the following content:
|
Create repository spec file dummies.repository.spec.js
inside app/dummies
with the following content:
|
Create integration file dummies.integration.js
inside app/dummies
with the following content:
|
We are now able to run npm test
and all unit tests and integration tests will kick off.
A nice coverage report for the unit and integration tests will be generate in de /coverage
folder.
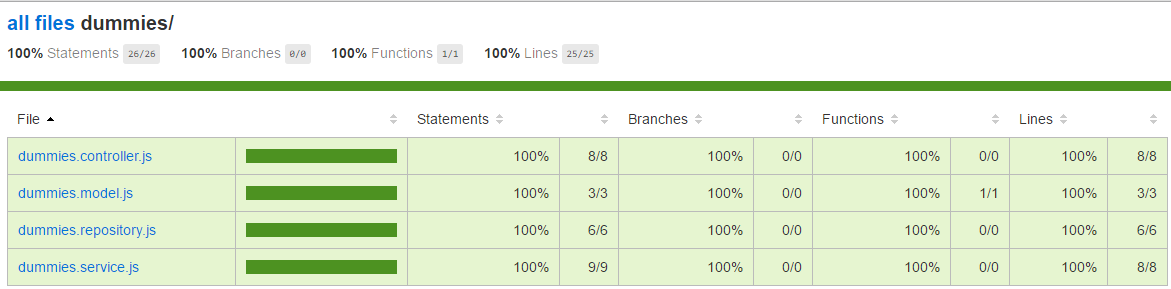
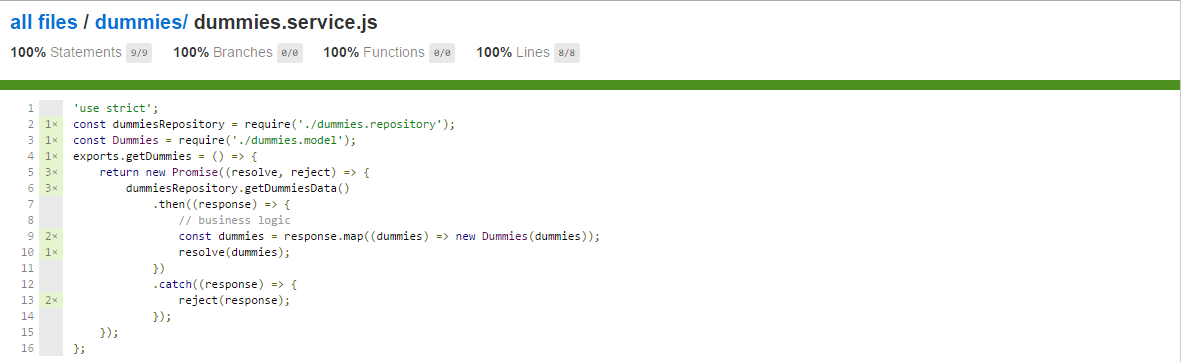
That’s it for this blog post! I will try to create a Yeoman template for this in the future. Feel free to leave feedback and comments or have any questions about this.